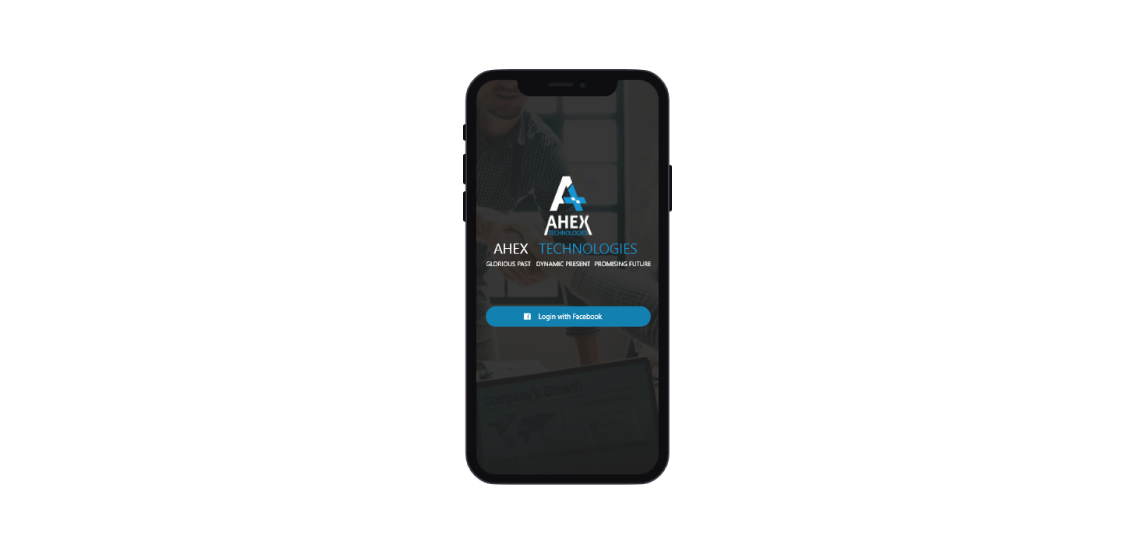
Introduction
Now a days we are using lots of web and mobile applications. Each application requires email and password for logging in and using the full feature of application.
With so many web/app accounts, the users struggle with remembering a strong password for all of their web/app accounts.
So to reduce the burden of remembering these many passwords, the developers got a clean and easy way for this sign in process. That is Social login.
Social login, provides users with one single login using an account they already have on different social networks like Facebook, Google+, Twitter etc. By using their Facebook, Twitter or Google+ account, users do not generate a new password or create a new account on a third-party website. Instead, they just use their social network login information.
There are several benefits of social login to users and developers. In this post I am going to explain how to Facebook login.
Advantages of using social login are :
- The biggest advantage is not having to build or implement your own authentication service.
- It gives users a simple, fast, and secure way to login to your app. No need for them to remember another username/password combination.
- Authenticity. For example, news websites have seen the quality of their article comments improve dramatically after implementing Facebook Login. No more trolling.
Is “login with Facebook” secure in an app?
Yes, it is completely secure because when you are using Facebook to login to some app , you are actually logging in to Facebook. Once your credentials are verified by Facebook server, you are redirected to the app meaning that login attempt was successful. Same happens with every other login that asks you to login via Google or some other account. So it is completely secure to login with Facebook.
Let’s talk about procedures for implementing login with Facebook.
How to implement Login with Facebook in your app?
1. Setting an App or Create a New App :
Go to this link https://developers.facebook.com/docs/facebook-login/ios here you need to create AppId. There you will get option between Search for your app or Create a New App.
Click on Create a New App. Add your display name and click on Create App ID.
2. Setup Development Environment :
Now for setting up development environment, best way is to use CocoaPods. Using CocoaPods
a.) Navigate to your project folder in terminal.
b.) Type the below commands
$ sudo gem install cocoapods
$ pod init $ open Podfile
Now in the Podfile paste pod ‘FBSDKLoginKit’ above “end” and save the Podfile.
$ pod install
After this come back to Facebook developers account and register Bundle ID of your app.
3. Register and configure your app with Facebook :
You need to register your bundel identifier (Bundle ID). In the box add Bundle ID from your app. Make Enable Single Sign-On YES.
4. Configure info.plist :
Most important is to configure info.plist file. Right-click info.plist, and choose Open As Source Code. On developers account you will be getting few lines of code to paste in info.plist file. Paste that code between <dict>…</dict>. Best is paste just above </dict>. e.g. snippet will look like below
5. Connect Your App Delegate :
Add the following code in your AppDelegate directly.
#import <FBSDKCoreKit/FBSDKCoreKit.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[[FBSDKApplicationDelegate sharedInstance] application:application didFinishLaunchingWithOptions:launchOptions];
// Add any custom logic here.
return YES;
}
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
BOOL handled = [[FBSDKApplicationDelegate sharedInstance] application:application openURL:url sourceApplication:options[UIApplicationOpenURLOptionsSourceApplicationKey] annotation:options[UIApplicationOpenURLOptionsAnnotationKey]
];
// Add any custom logic here.
return handled;
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[[FBSDKApplicationDelegate sharedInstance] application:application didFinishLaunchingWithOptions:launchOptions];
// Add any custom logic here.
return YES;
}
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
BOOL handled = [[FBSDKApplicationDelegate sharedInstance] application:application openURL:url sourceApplication:options[UIApplicationOpenURLOptionsSourceApplicationKey] annotation:options[UIApplicationOpenURLOptionsAnnotationKey]
];
// Add any custom logic here.
return handled;
}
// Below is required when iOS version is less than iOS 10
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)url sourceApplication:(NSString *)sourceApplication annotation:(id)annotation {
BOOL handled = [[FBSDKApplicationDelegate sharedInstance] application:application openURL:url sourceApplication:sourceApplication annotation:annotation
];
// Add any custom logic here.
return handled;
}
6. Add Facebook Login to Your Code :
In your view controller add the following code
// ViewController.m
// LoginWithFacebookDemo
//
// Created by Admin on 28/04/18.
// Copyright © 2018 Ahex Technologies. All rights reserved.
//
#import "ViewController.h"
#import <FBSDKCoreKit/FBSDKCoreKit.h>
#import <FBSDKLoginKit/FBSDKLoginKit.h>
#import "SecondViewController.h"
#import "ModelClass.h"
@interface ViewController () {
CGRect rect;
}
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
rect = [[UIScreen mainScreen] bounds];
UIButton *facebookButton = [[UIButton alloc]initWithFrame:CGRectMake(12, rect.size.height-60-24, rect.size.width-24, 60)];
facebookButton.layer.cornerRadius = 5.0;
[facebookButton setBackgroundColor:[UIColor blueColor]];
[facebookButton addTarget:self action:@selector(facebookButtonAction) forControlEvents:UIControlEventTouchUpInside];
[facebookButton setTitle:@"Login With Facebook " forState:UIControlStateNormal];
[facebookButton setTitleColor:[UIColor whiteColor] forState:UIControlStateNormal];
[self.view addSubview:facebookButton];
}
-(void)facebookButtonAction {
if ([FBSDKAccessToken currentAccessToken]) {
// User is logged in, do work such as go to next view controller.
NSLog(@"Current Token Value:%@",FBSDKAccessToken.currentAccessToken);
SecondViewController *settings_obj = [[UIStoryboard storyboardWithName:@"Main" bundle:nil]instantiateViewControllerWithIdentifier:@"SecondViewController"];
[self.navigationController pushViewController:settings_obj animated:YES];
}
else
{
// Take user to login with facebook page
FBSDKLoginManager *login = [[FBSDKLoginManager alloc] init];
[login
logInWithReadPermissions: @[@"public_profile", @"email", @"user_friends"]
fromViewController:self
handler:^(FBSDKLoginManagerLoginResult *result, NSError *error) {
if (error)
{
//NSLog(@"Process error");
}
else if (result.isCancelled)
{
//NSLog(@"Cancelled");
}
else
{
NSMutableDictionary* parameters = [NSMutableDictionary dictionary];
[parameters setValue:@"email,first_name,last_name,picture.type(large),gender,verified,id" forKey:@"fields"];
[[[FBSDKGraphRequest alloc] initWithGraphPath:@"me" parameters:parameters]
startWithCompletionHandler:^(FBSDKGraphRequestConnection *connection,
id result, NSError *error) {
if (!error)
{
NSLog(@"Value of result is:%@",result);
NSString *emailObject = [NSString stringWithFormat:@"%@", [result objectForKey:@"email"]];
NSString *idObject = [NSString stringWithFormat:@"%@", [result objectForKey:@"id"]];
NSString *firstNameObject = [NSString stringWithFormat:@"%@", [result objectForKey:@"first_name"]];
NSString *lastNameObject = [NSString stringWithFormat:@"%@", [result objectForKey:@"last_name"]];
NSString *gender = [NSString stringWithFormat:@"%@", [result objectForKey:@"gender"]];
NSString *verifiedObject =@"true";
NSDictionary *fbDict = @{
@"verified":verifiedObject,
@"fb_user_id":idObject,
@"first_name":firstNameObject,
@"last_name":lastNameObject,
@"gender":gender,
@"email":emailObject
};
NSLog(@"Value of facebookDict is:%@",fbDict);
ModelClass.sharedInstance.userProfileDictionary = fbDict;
}
}]; // parameters
SecondViewController *svc = [[UIStoryboard storyboardWithName:@"Main" bundle:nil]instantiateViewControllerWithIdentifier:@"SecondViewController"];
[self.navigationController pushViewController:svc animated:YES];
}// else
}]; // login
} // else
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
7. Remove the Facebook access token :
For Log out you need to add the following code to the logout button
Put below code in SecondViewController.h
@interface SecondViewController : UIViewController
- (IBAction)logoutAction:(UIButton *)sender;
@property (strong,nonatomic) NSDictionary *userDataDict;
@property (strong, nonatomic) IBOutlet UILabel *dictionaryLabel;
@end
Put below code in SecondViewController.m
// SecondViewController.m
// LoginWithFacebookDemo
//
// Created by Admin on 28/04/18.
// Copyright © 2018 Ahex Technologies. All rights reserved.
//
#import "SecondViewController.h"
#import <FBSDKLoginKit/FBSDKLoginKit.h>
#import "ModelClass.h"
@interface SecondViewController ()
@end
@implementation SecondViewController
- (void)viewDidLoad {
[super viewDidLoad];
_userDataDict = ModelClass.sharedInstance.userProfileDictionary;
_dictionaryLabel.text = [NSString stringWithFormat:@"%@",_userDataDict];
// Do any additional setup after loading the view.
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)logoutAction:(UIButton *)sender {
FBSDKLoginManager *login = [[FBSDKLoginManager alloc] init];
[login logOut];
UIAlertController *alert = [UIAlertController alertControllerWithTitle:@"Success" message:@"logged out successfully" preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *okAction = [UIAlertAction actionWithTitle:@"OK" style:UIAlertActionStyleDefault handler:^(UIAlertAction * action)
{
// Ok action example
[self.navigationController popViewControllerAnimated:YES];
}];
UIAlertAction *otherAction = [UIAlertAction actionWithTitle:@"Cancel" style:UIAlertActionStyleDefault handler:^(UIAlertAction * action)
{
// Other action
//[self.navigationController popViewControllerAnimated:YES];
}];
[alert addAction:okAction];
[alert addAction:otherAction];
[self presentViewController:alert animated:YES completion:nil];
}
@end
8.Modal Class :
I have used modal class for storing the dictionary of user information and displaying it in SecondViewController.
ModelClass.h
// ModelClass.h
// ModelDemo
//
// Created by admin on 20/04/18.
// Copyright © 2018 Ahex Technologies. All rights reserved.
//
#import <Foundation/Foundation.h>
@interface ModelClass : NSObject
@property (strong, nonatomic) NSDictionary *userProfileDictionary;
+ (instancetype)sharedInstance;
@end
ModelClass.m
//
// ModelClass.m
// ModelDemo
//
// Created by admin on 20/04/18.
// Copyright © 2018 Ahex Technologies. All rights reserved.
//
#import "ModelClass.h"
@implementation ModelClass
-(instancetype)init {
self=[super init];
if (self)
{
}
return self;
}
+ (instancetype)sharedInstance {
static ModelClass *sharedInstance = nil;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
sharedInstance = [[ModelClass alloc] init];
// Do any other initialisation stuff here
});
return sharedInstance;
}
@end
Summary
So here we can see how easily we can integrate Facebook login feature to our app. Also it will reduce the burden of creating your own login and authentication system. We could easily authenticate the user and allow them to use the great features provided by our app.
If this article is helpful then do share. If you want some more information or feel any difficulty while integrating Facebook login, please tell in comment. And feedbacks are always welcome.