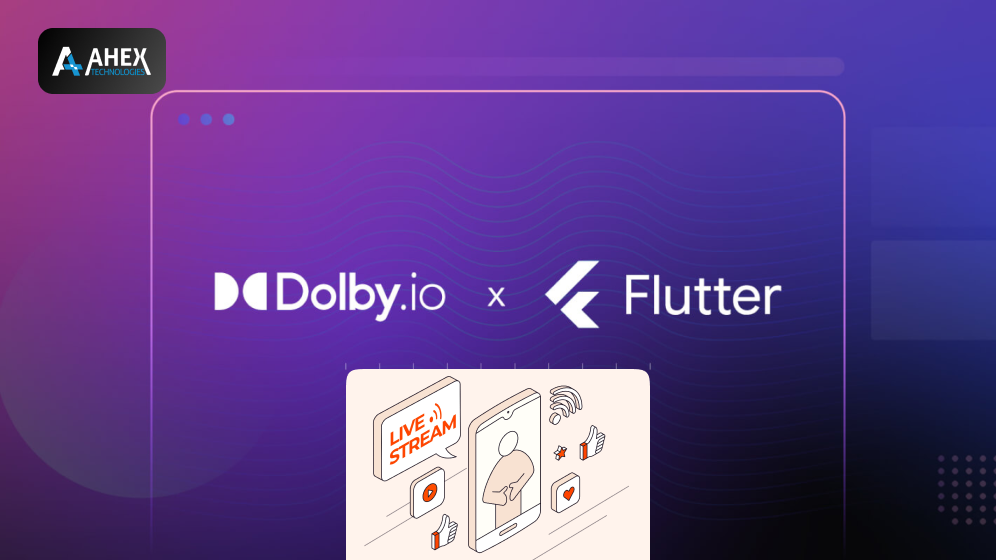
Real-time streaming has become a cornerstone of modern mobile applications, enabling everything from live video broadcasts to interactive gaming experiences. One of the most powerful combinations for creating these applications is using Flutter, Google’s open-source UI toolkit, alongside Dolby.io’s real-time streaming services. In this guide, we will walk through the process of building a real-time streaming app with Flutter and Dolby.io, ensuring you can create robust and engaging applications with minimal hassle.
Why Choose Flutter and Dolby.io for Real-Time Streaming?
Flutter’s Advantages:
- Cross-Platform Development: Write once, deploy on iOS and Android.
- Rich UI Components: Build beautiful interfaces with ease.
- High Performance: Near-native performance for demanding applications.
- Strong Community Support: Access to a vast ecosystem of plugins and resources.
Dolby.io’s Advantages:
- High-Quality Audio and Video: Industry-leading streaming quality.
- Scalability: Easily handle thousands of concurrent users.
- Comprehensive SDKs: Simple integration with robust functionalities.
- Low Latency: Real-time interactions with minimal delay.
- Step 1: Setting Up Your Development Environment\
Install Flutter:
First, ensure you have Flutter installed on your system. You can download it from Flutter’s official website.
bash
Copy code
$ flutter doctor
Run the flutter doctor command to check if Flutter is correctly installed and if all necessary dependencies are resolved.
Set Up Dolby.io Account:
Sign up for a Dolby.io account and get your API keys. You can sign up at Dolby.io.
Step 2: Creating a New Flutter Project
Create a new Flutter project by running:
bash
Copy code
$ flutter create realtime_streaming_app
$ cd realtime_streaming_app
Open the project in your preferred IDE.
Step 3: Adding Necessary Dependencies
Add Flutter Plugins:
Open pubspec.yaml and add the necessary dependencies for your project.
yaml
Copy code
dependencies:
flutter:
sdk: flutter
provider: ^6.0.0
http: ^0.13.4
# Add any other necessary dependencies
Add Dolby.io SDK:
Download the Dolby.io Flutter SDK from their official GitHub repository and add it to your project. Include the necessary configurations as per the Dolby.io documentation.
Step 4: Setting Up Dolby.io SDK
Initialize the SDK:
In your main.dart, initialize the Dolby.io SDK with your API keys.
dart
Copy code
import 'package:flutter/material.dart';
import 'package:dolbyio/dolbyio.dart'; // hypothetical import, replace with actual package name
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Dolbyio.initialize('YOUR_API_KEY');
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Real-Time Streaming App',
home: HomePage(),
);
}
}
Step 5: Building the User Interface
Create a Home Page:
Create a simple UI for the home page where users can start streaming.
dart
Copy code
import 'package:flutter/material.dart';
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Real-Time Streaming'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => StreamingPage()),
);
},
child: Text('Start Streaming'),
),
),
);
}
}
Create a Streaming Page:
This page will handle the real-time streaming functionalities.
dart
Copy code
import 'package:flutter/material.dart';
import 'package:dolbyio/dolbyio.dart'; // hypothetical import, replace with actual package name
class StreamingPage extends StatefulWidget {
@override
StreamingPageState createState() => StreamingPageState();
}
class _StreamingPageState extends State<StreamingPage> {
@override
void initState() {
super.initState();
startStreaming();
}
void startStreaming() async {
await Dolbyio.startStreaming();
// Handle stream logic here
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Live Streaming'),
),
body: Center(
child: Text('Streaming...'),
),
);
}
}
Step 6: Handling User Permissions
For real-time streaming, you’ll need to request permissions to access the camera and microphone. Update your AndroidManifest.xml and Info.plist files accordingly.
Android:
Add the following permissions to AndroidManifest.xml:
xml
Copy code
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
iOS:
Add the following keys to Info.plist:
xml
Copy code
<key>NSCameraUsageDescription</key>
<string>We need to use your camera for live streaming.</string>
<key>NSMicrophoneUsageDescription</key>
<string>We need to use your microphone for live streaming.</string>
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
Step 7: Implementing Real-Time Features
Chat Functionality:
Add chat functionality to allow users to interact in real-time during the stream.
dart
Copy code
class ChatWidget extends StatelessWidget {
final TextEditingController _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return Column(
children: [
Expanded(
child: ListView(
children: [
// Display chat messages here
],
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
children: [
Expanded(
child: TextField(
controller: _controller,
decoration: InputDecoration(
hintText: 'Enter your message...',
),
),
),
IconButton(
icon: Icon(Icons.send),
onPressed: () {
// Send message logic here
_controller.clear();
},
),
],
),
),
],
);
}
}
Viewer Count:
Display the number of viewers in real-time.
dart
Copy code
class ViewerCountWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return StreamBuilder<int>(
stream: Dolbyio.viewerCountStream,
builder: (context, snapshot) {
return Text(
'Viewers: ${snapshot.data ?? 0}',
style: TextStyle(fontSize: 18),
);
},
);
}
}
Step 8: Testing and Debugging
Test on Multiple Devices:
Ensure your app works seamlessly across various devices and screen sizes.
Debugging:
Use Flutter’s debugging tools and Dolby.io’s dashboard to monitor and troubleshoot any issues.
Step 9: Deploying Your App
Prepare for Deployment:
Before deploying, make sure to optimize your app for performance and security.
Publish on App Stores:
Follow the guidelines for publishing apps on the Google Play Store and Apple App Store.
Conclusion
Building a real-time streaming app with Flutter and Dolby.io is a powerful way to leverage modern technology to create engaging and interactive user experiences. By following this step-by-step guide, you can develop a robust application that combines Flutter’s versatile UI toolkit with Dolby.io’s high-quality streaming services. Whether you are building a social media platform, a live event broadcasting app, or an interactive gaming experience, this combination offers the tools and capabilities needed to succeed.
In summary, the integration of Flutter and Dolby.io can significantly enhance the capabilities of your app, providing users with a seamless and high-quality streaming experience. As real-time streaming continues to grow in popularity, staying ahead of the curve with the right technology stack will be crucial for any developer or business looking to create cutting-edge applications.