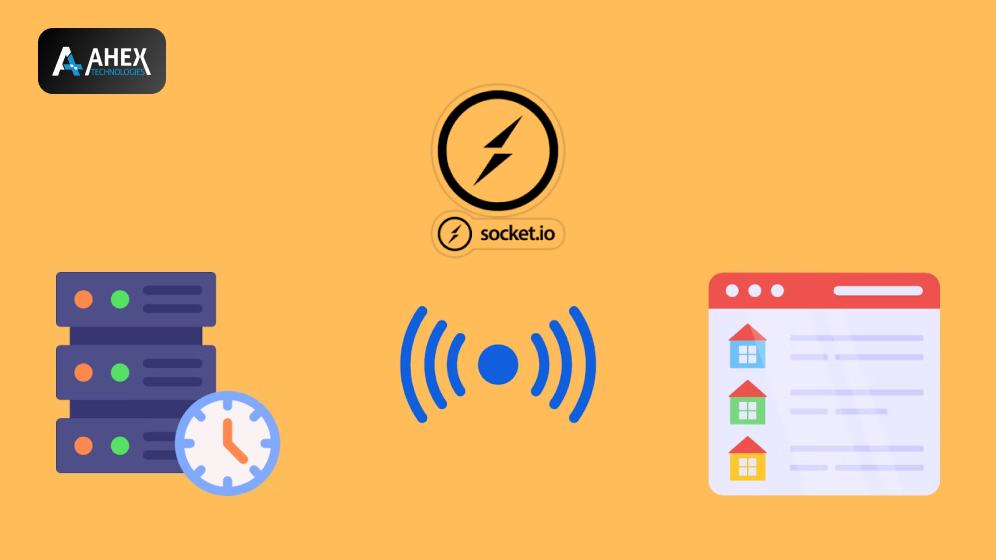
Definition
Socket.IO is a library that enables low-latency, bidirectional and event-based communication between a client and a server. It is designed to work seamlessly across various platforms and browsers, ensuring a reliable connection even in environments with network issues or firewalls. Socket.IO abstracts away the complexities of WebSockets, making it easier for developers to build real-time applications with minimal effort.
This guarantees the delivery provided by the underlying TCP connection.
Socket.IO follows an event-driven architecture, where communication between the server and client happens through events. There are two key types of events:
Emitter events and Listener events.
- Emitter events: These events send (emit) data from one side (client or server) to the other. For example, a client can emit a message to the server, or the server can emit a notification to a client.
Ex:- socket.emit(‘message’, ‘Hello, server!’);
Here ‘message’ is the name of the event and ‘Hello, server!’ is data sent through an event.
- Listener Events: These are the events that wait (listen) for incoming data from an emitter. When an event is emitted, the listener receives it and executes the callback function.
Ex:- socket.on(‘message’, (data) => { console.log(‘Received message:’, data); });
Here on what name we send the event. From the other side(client or server) on the same name we need to get the event.
Rooms: A server-side-only concept that lets you create a room inside which you can listen to or emit events from multiple sources. Clients can join or leave rooms, allowing the server to send messages to multiple clients at once.
Ex: – socket.join(‘chatRoom1’);
Ex: – io.to(‘chatRoom1’).emit(‘message’, ‘Hello everyone in the room!’);
Advantages
The Socket.IO connection can be established with different low-level transports:
- HTTP long-polling
- Long-polling is a technique where the client requests data from the server and the server holds the request open until new data becomes available. When new data is sent, the connection closes and the client immediately sends another request. This method mimics real-time communication over HTTP but has higher latency compared to WebSockets.
- WebSocket is a protocol that enables full-duplex communication channels over a single TCP connection. Unlike HTTP, WebSockets allow continuous two-way communication between the client and server, reducing overhead and latency for real-time data exchange.
- WebTransport is a modern web API that enables low-latency and secure transmission of data between client and server, particularly useful for real-time applications. It is built on top of the HTTP/3 protocol and supports both unidirectional and bidirectional streams, as well as datagram messages.
- Underlying TCP
- TCP (Transmission Control Protocol) is a core protocol of the Internet Protocol Suite, ensuring reliable, ordered, and error-checked delivery of data between client and server. All the above transports (HTTP long-polling, WebSocket, and WebTransport) are built on top of TCP, providing a solid foundation for reliable communication.
Socket.IO will automatically pick the best available option.
Features
Here are the features provided by Socket.IO over plain WebSockets:
- Automatic reconnection
- Socket.IO automatically attempts to reconnect the client to the server when the connection is lost due to network issues or server restarts. It includes customizable reconnection attempts and delays to ensure a reliable connection, whereas plain WebSockets require manual implementation for reconnection logic.
- Packet buffering
- In cases where the connection is temporarily lost, Socket.IO buffers packets (messages) on the client side until the connection is re-established. Once the connection is restored, the buffered messages are sent to the server. WebSockets, on the other hand, would drop messages during the disconnection unless explicitly handled by the developer.
- Acknowledgments
- Socket.IO allows for acknowledgments (callbacks) when a message is successfully received by the recipient (client or server). This enables confirmation of message delivery, which plain WebSockets don’t provide natively without additional coding for handling delivery guarantees.
- Broadcasting
- Broadcasting refers to the ability to send a message from one client or server to multiple clients at once. Socket.IO makes it easy to broadcast messages to all connected clients or specific groups (rooms). Plain WebSockets require more complex logic to achieve broadcasting as it’s not built into the protocol.
- Multiplexing
- Multiplexing in Socket.IO allows multiple independent communication channels (namespaces) to be established over a single WebSocket connection. This enables separating different types of events or rooms logically. Plain WebSockets do not support multiplexing, and developers would need to open multiple WebSocket connections for different functionalities.
Difference b/w sockets vs API
Socket.IO allows for real-time, bidirectional communication between the client and server. This means that both the client and server can send and receive data instantly, without needing to explicitly request it. Which means, Socket.IO maintains a persistent connection between the client and server using WebSockets or fallback technologies, ensuring low-latency communication. But, Request-Response Model Traditional APIs follow a request-response model where the client sends a request to the server, and the server returns a response. Each request is independent of the others, and the server doesn’t retain any session information between requests. Server has to make another connection for every API Request.
Supported Languages
Client Side
- Javascript (Node js, React js, Angular js, React Native)
- Java
- C++
- Swift
- Dart(flutter)
- Python
- .net
- Kotlin
- PHP
Server Side
- Javascript (Node js)
- Java
- Python
- GoLang
- Rust
Real world Examples
- Live Cricket Score (in google, cricbuzz etc.).
- Real world chat applications ( whatsapp, facebook messenger).
- Real time notifications.
- Collaborative tools like (google docs, figma etc).
- Real world room based gaming applications ( pubg, free fire).
- Live Stock Market Updates.
Conclusion:
With Socket.IO, you don’t need to refresh your page to get updates like you would with regular API calls. Once a connection is made, Socket.IO keeps it open, allowing real-time communication for messages or notifications without any extra effort. This makes it perfect for chat apps, live updates, online games, or any situation where you need instant data updates between a client and server. It simplifies the process of building real-time features, ensuring a smooth and efficient experience for users.
Prerequisite: choose your frontend or backend language and do project setup.
Install dependencies: (in your preferred languages)
socket.io for server side implementation
socket.io-client for client side implementation.
Related Links
https://admin.socket.io/#/sockets
Sample Code
1) how to connect to socket.io server (client and server code) in Nodejs.
2) how to emit an event with sockets in node js
3) how to join rooms and send messages to rooms in node js.